In programming, data types are fundamental concepts that determine what types of data can be used in a program and how they can be processed.
Data types in programming are defined using the programming language you use to write code.
There are several basic types of data used in programming.
In this article, we'll look at the most common types and how they're used in various programming languages.
Integer data types
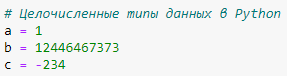
Integer data types are used to store integers without a fractional part. They may be iconic or unfamiliar. Signed integer data types can store negative numbers, while unsigned integer data types can only store positive numbers.
For example, in the C programming language, the integer type “int” is used to store integers. In Python, integer types can be defined using the “int” keyword.
Floating-point data types
.png)
Floating-point data types are used to store fractional numbers. They can also be iconic or unsigned.
For example, in the C programming language, the “float” data type is used to store single-precision floating-point numbers, and the “double” data type is used to store double-precision floating-point numbers. In Python, you can define a floating-point data type using the “float” keyword.
Symbolic data types

Symbolic data types are used to store characters such as letters, numbers, and punctuation marks.
For example, in the C programming language, the character data type “char” is used to store a single character. In Python, a symbolic data type can be defined using the “str” keyword.
Logical data types

Logical data types are used to store truth values that can be either true or false.
For example, in the C programming language, the logical data type “bool” is used to store truth values. In Python The logical data type is also “bool” and can take True or False values.
Logical data types are widely used in programming to make decisions based on conditions. For example, you can use a logical data type to check whether a number is even or odd. If the number is even, the logical data type will be true; if the number is odd, it will be false.
Arrays

Arrays are a data type that can contain multiple values of the same type. Arrays are used to store multiple values that can be processed together.
For example, in the C programming language, an array can be defined as follows:
int numbers [5] = {1, 2, 3, 4, 5};
This is the definition of the “numbers” array, which contains 5 integer values. In Python, arrays can be defined using the “list” data structure.
Data structures
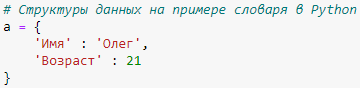
Data structures are data types that combine several values of different types into a single structure. Data structures are used for more complex data operations, such as storing information about a user, items in a store, or contacts in an address book.
For example, in the C programming language, the data structure can be defined as follows:
struct Person {
char name [50];
int age;
float salary;
};
This is the definition of a “Person” data structure, which contains name (string value), age (integer value), and salary (floating-point value).
Classes
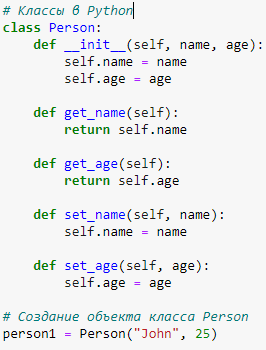
Classes are templates for creating objects that can contain data and methods for processing it. Classes are a fundamental concept in object-oriented programming and are used to model real objects or processes.
Classes can contain variables called fields and methods that can be used to perform actions on those fields. For example, the “Student” class may contain the fields “first name”, “last name”, and “grades”, and the “GPA” method can be used to calculate a student's GPA.
Reference data
.png)
Reference data is data that is stored in memory and that variables reference. Unlike primitive data types like numbers and symbols, reference data is not stored directly in the variable. Instead, the variable contains a reference to an object in memory.
Reference data can be used to create complex data structures such as lists, trees, and graphs. They also allow you to create objects from the classes we talked about earlier.
Typing
.png)
Typing is the process of determining the data type of a variable in a program. Typing can be static or dynamic. In static typing, the variable type is determined at compile time, and in dynamic typing, the type is determined at program execution time.
Static typing can help prevent program errors associated with the misuse of data types, but requires more careful work during the development process. Dynamic typing is more flexible, but it can lead to errors due to improper use of data types.
In conclusion, data types are fundamental concepts in programming that allow us to store and process different types of data.
Different programming languages may have their own data types, but the basic concepts remain the same.
Understanding data types and using them is important for creating effective and reliable programs. Incorrect use of data types can lead to execution errors, which will make the program's output unpredictable.
In addition, using data types correctly can help speed up program execution, reduce memory consumption, and improve program performance.
Well, if you want to learn how to code in Python, sign up for a free trial lesson in our ProgKids programming school for children.
Karen Konstantin
Progkids teacher